Kernel also manages process address space.
Linux is a virtual memory operating system.
Virtual memory technique enables operating system to execute a program which is larger than the physical memory.
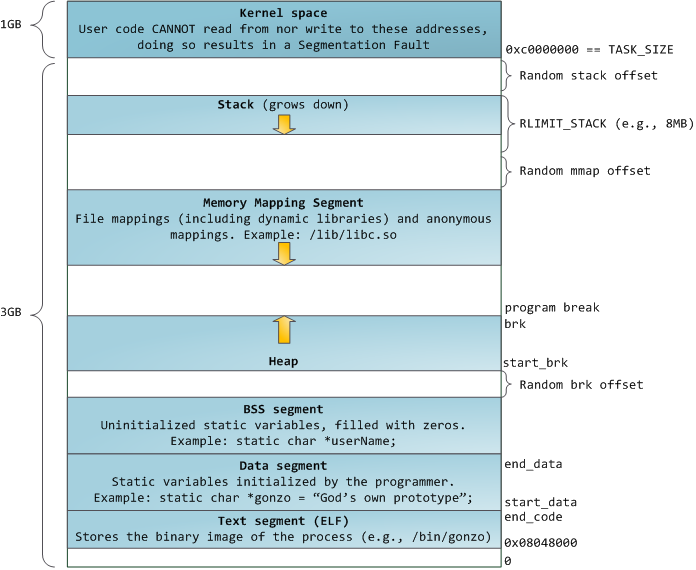
Kernel thread do not have process address space, hence no memory descriptor. Even kernel threads require page table to access kernel memory.
How does kernel threads work ???
Kernel space is constantly present (never gets swapped out) and maps the same physical memory in all processes
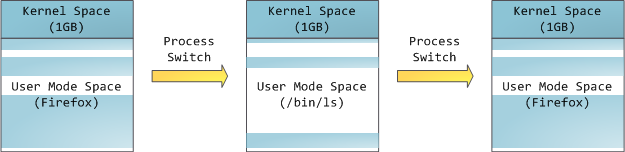
Linux kernel divides the process address space into Virtual Memory Area (VMA). Each VMA can have unique access permissions.
If a process try to access memory address not in a valid memory area the kernel kills the process with the dreaded “Segmentation Fault” message.
The Linux kernel maintains a private page table for each program.
Each process has its own page table (threads share them).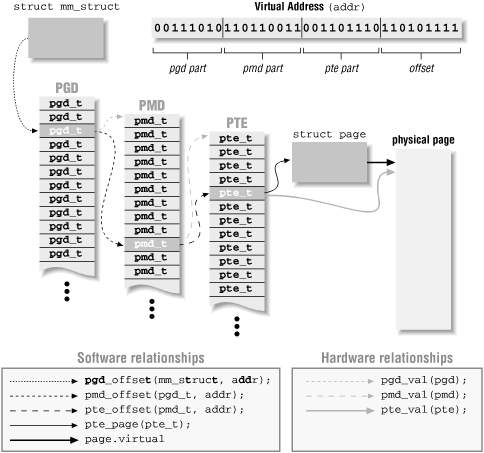

mmap() creates a new VMA.
------------start new.c-----------size new
#include <stdio.h>
#include <fcntl.h>
#include <sys/mman.h>
int main()
{
char buffer [200] = {};
char filename [] = "test.txt";
char buf [] = "HELLO WORLD!!!\n";
int file;
void *target;
if((file = open(filename, O_RDWR|O_CREAT, 0777)) == -1)
fprintf(stderr, "%s: Error: opening file: %s\n", \
"mmap", filename), exit(1);
lseek(file, strlen(buf) - 1, SEEK_SET);
write(file, '\0', 1);
if((target = mmap(0, strlen(buf), PROT_READ|PROT_WRITE, \
MAP_SHARED, file, 0)) == (void *) -1)
fprintf(stderr, "Error mapping input file: %s\n", filename), exit(1);
memcpy(target, buf, strlen(buf));
sprintf(buffer, "cat /proc/%d/maps", getpid());
printf("%s\n", system(buffer));
munmap(target, strlen(buf));
return 0;
}
----------------------------------
gcc –o new new.c
./new
---------------output-------------
08048000-08049000 r-xp 00000000 03:03 65519 /home/humble/new
08049000-0804a000 rw-p 00000000 03:03 65519 /home/humble/new
0804a000-0804b000 rwxp 00000000 00:00 0
40000000-40013000 r-xp 00000000 03:02 292049 /lib/ld-2.x.so
40013000-40014000 rw-p 00013000 03:02 292049 /lib/ld-2.x.so
40014000-40015000 rw-s 00000000 03:03 65707 /home/humble/test.txt
42000000-4212c000 r-xp 00000000 03:02 308273 /lib/i686/libc-2.x.so
4212c000-42131000 rw-p 0012c000 03:02 308273 /lib/i686/libc-2.x.so
42131000-42135000 rw-p 00000000 00:00 0
bfffd000-c0000000 rwxp ffffe000 00:00 0
----------------------------------
strip new
ldd new
readelf –a new
Reference:
http://blog.chinaunix.net/u2/70942/showart_1227245.html
http://www.c.happycodings.com/Gnu-Linux/code6.html